Web Components
Unit uses web components to allow simple integration that is platform and framework agnostic. The components work seamlessly on both desktop and mobile, and support all modern browsers.
Web components run in a shadow DOM, which encapsulates Unit Code from your app and makes sure the components don't impact the behavior of the host app, and vice versa.
The project is being built with performance and accessibility in mind, and complies with modern standards such as Core Web Vitals. The components are covered by end-to-end automated tests.
Installation
Import the Unit Web SDK by adding a <script>
tag at the head of your HTML page.
For production use:
<script async src="https://ui.unit.co/release/latest/components.js"></script>
For sandbox use:
<script async src="https://ui.s.unit.sh/release/latest/components.js"></script>
Versioning
The components.js
file follows a semantic versioning numbering scheme.
Our library and all of its versions are hosted on our CDN and are accessible on https://ui.s.unit.sh/release/X.Y.Z/components.js
for sandbox or https://ui.unit.co/release/X.Y.Z/components.js
for production.
Version Resolution Strategy
The latest version is available on https://ui.s.unit.sh/release/latest/components.js or https://ui.unit.co/release/latest/components.js, and the version number will appear on the first rows of the file with the following text:
* @license Unit White-Label UI Components
* unit-web-sdk X.Y.Z
While it's possible to work with the latest version, developers may choose to use a specific one, or by using one of the following ways:
It's possible to fetch the recent version of a specific minor by specifying the minor version only (X.Y). for example: if we had versions 1.0.0, 1.0.1, 1.1.0, 1.2.3 and the script src that was defined is
https://ui.s.unit.sh/release/1.0/components.js
, the file version that will be returned is 1.0.1.In the same manner, it's possible to fetch the recent version of a specific major by specifying the major version only (X). for example: if the latest version is 1.2.3 and the script src that was defined is
https://ui.s.unit.sh/release/1/components.js
, the file version that will be returned is 1.2.3.
This allows developers a flexible approach for receiving updates, bug fixes and design adjustments, but also enables a way to use a specific version, and opt-in to newer versions later.
ⓘ NOTE
We recommend specifying the latest minor version (for example:
1.2
) and leaving out the last part of the version to make sure you get the latest patch and bug fixes.
Release Cycle
Our release cycle allows for frequent patch updates for bug fixes and internal improvements, UI changes are to be avoided on these versions.
Minor versions will be released every week or two and will include any non-breaking change to current components, or a release of a new component. New flows for current components may be introduced, and a web-component attribute (allow-new-component-feature="true"
) will be introduced to allow developers to opt-in to using it.
Major versions are seldom released and their changes are planned and announced ahead of time, these may introduce breaking changes or a major UI revamp.
Authentication
The web components uses Unit customer tokens as a way to verify the customer identity.
Customer token can be set as an attribute on each component, or can be set globally for all components under the key unitCustomerToken
in Local Storage.
Events
The web components fire Custom events to let you know of any customer activity taking place within each component.
The events can be used for:
- Having your app react to changes originating from the component (for instance, if a card gets closed, you may want to redirect the client back to the home screen).
- Managing your own analytics on customer usage patterns.
- Sending commands to the component (for instance, you can tell the card component to freeze the card by sending an event).
Web components that interact with the Unit API will emit custom events to the window object upon completion of any HTTP requests. These events come with a promise object that represents the outcome of the request. The promise will be resolved if the request is successful, providing access to the response data. If the request fails, the promise will be rejected and the error can be handled through the catch method. This feature allows for efficient handling of the request-response cycles within the web component, without having to manually handle the promises returned by the API.
window.addEventListener("unitCardStatusChanged", async function (e) {
e.detail
.then(function (data) {
console.log(data);
})
.catch((e) => {
console.log(e);
});
});
On load event
The unitOnLoad event is a custom event that is fired by the web component once it has finished loading and is ready to be used. In order to listen for this event, you need to add an event listener to the desired component using the addEventListener
method.
The event listener function will be called with an event object as its parameter, which contains information about the event.
document
.querySelector("unit-elements-card[card-id='632197']")
.addEventListener("unitOnLoad", function (e) {
console.log("Component loaded", e.detail);
});
Error handling
In case of an error during the loading process, the onload event will be fired with an error message. The error message will be passed as the detail property of the event object.
document
.querySelector("unit-elements-card[card-id='632197']")
.addEventListener("unitOnLoad", function (e) {
if (e.detail.errors) {
console.error("Error loading component: ", e.detail.errors);
}
});
For example, if a customer's customer token has expired, it is necessary for your site or app to re-authenticate the current customer.
document
.querySelector("unit-elements-account")
.addEventListener("unitOnLoad", function (e) {
if (e.detail.errors && e.detail.errors[0].status === "401") {
console.error("Customer token has expired, please re-authenticate");
// Your site or app's custom code for re-authenticating a Unit customer
}
});
Check out the error handling documentation to learn more about Unit API errors structure.
Request refresh event
The unitRequestRefresh event can be used in order to refresh the web component
document
.querySelector("unit-elements-card[card-id='632197']")
.dispatchEvent(new CustomEvent("unitRequestRefresh"));
Components working together
Unit web components within the same document can effectively communicate with each other. They can automatically exchange messages and trigger events to update relevant data in other components.
For instance, the Payment components and Activity components can work together such that, after a payment has been processed, the new transactions are automatically displayed on the Activity component.
Similarly, the Payment components and Account components can communicate such that, after a payment has been made, the account balance is updated on the Account component.
In some cases, a component may need to be updated in response to an event that is triggered by another component.
For example, if a customer has multiple accounts, the app may have an Account component and an Activity component that displays the activity of a selected account.
<unit-elements-account account-id="1105561"></unit-elements-account>
<unit-elements-activity account-id="1105561"></unit-elements-activity>
The Account component has an account switcher that can change the current selected account.
By listening to the Account component's unitAccountChanged event, a change to the Activity component can be triggered to display the new account activity.
To accomplish this, the following code snippet can be used:
document
.querySelector("unit-elements-account")
.addEventListener("unitAccountChanged", async function (e) {
const eventData = await e.detail;
const selectedAccountId = eventData.data.id;
document
.querySelector("unit-elements-activity")
.setAttribute("account-id", selectedAccountId);
});
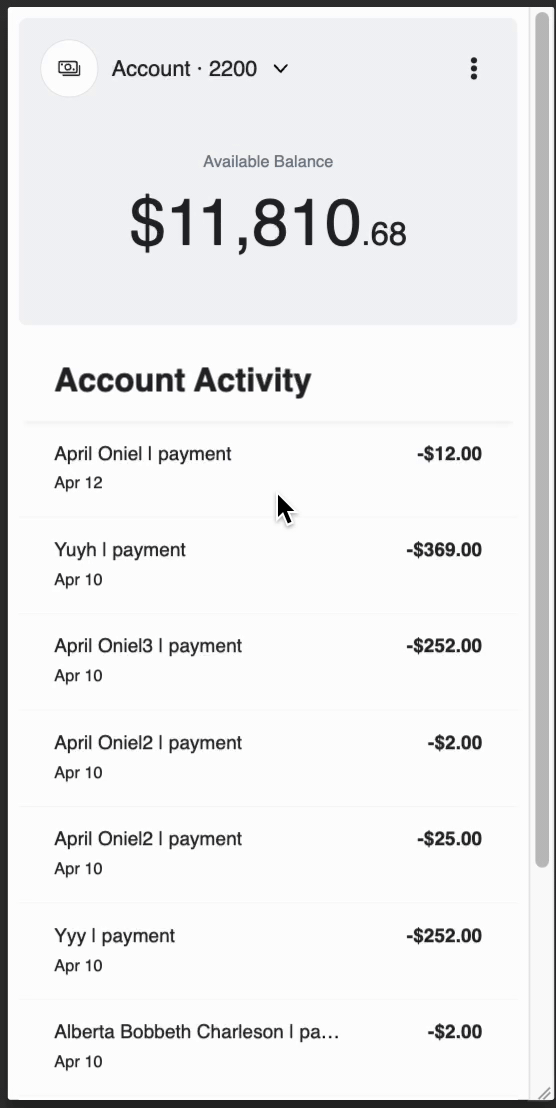
Card Component
Implementation
Add the card element to your app where you'd like the card to be presented.
<unit-elements-card
card-id="632197"
customer-token=""
theme=""
learn-more-url="www.google.com"
menu-items="freeze,addToWallet,managePin,replace,report,close"
></unit-elements-card>
<!-- Hide actions menu button & sensitive data button. -->
<unit-elements-card
card-id="632197"
customer-token=""
theme=""
hide-actions-menu-button="false"
hide-sensitive-data-button="false"
></unit-elements-card>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
card-id | string | Yes | Unit card id. |
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers cards cards-sensitive cards-sensitive-write cards-write |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
hide-actions-menu-button | string | No | Hide menu button in case value is 'true'. |
hide-card-title | string | No | Hide card title in case value is 'true'. |
hide-sensitive-data-button | string | No | Hide sensitive data button in case value is 'true'. |
learn-more-url | string | No | A “Learn more” URL on the report lost/close card info note. |
menu-items | string | No | A list of actions, The menu dynamically adjusts based on the provided list freeze,addToWallet,managePin,replace,report,close . |
Events:
Event Name | Description | Detail |
---|---|---|
unitOnLoad | Occurs when a component is loaded | Card |
unitCardStatusChanged | Occurs when a Card status changes, excluding first time card activation | [Promise] Card |
unitCardActivated | Occurs when a Card is activated | [Promise] Card |
Incoming events:
In some cases, the default menu button won't fit into the design of an application. By using the unitRequestOpenActionsMenu
incoming event, it's possible to open the card actions bottom sheet from a custom button
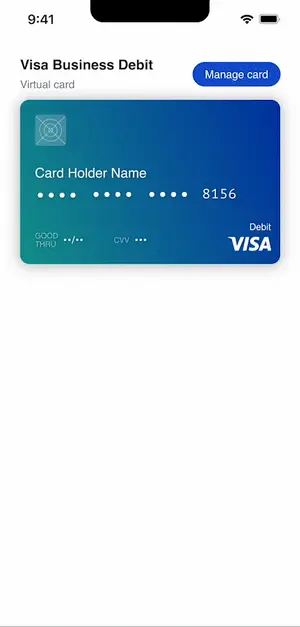
<unit-elements-card
hide-actions-menu-button="false"
hide-card-title="false"
hide-sensitive-data-button="false"
card-id="632197"
>
</unit-elements-card>
<button id="#custom-button">Manage Card</button>
<script>
document.querySelector("#custom-button").addEventListener("click", () => {
document
.querySelector("unit-elements-card")
.dispatchEvent(new CustomEvent("unitRequestOpenActionsMenu"));
});
</script>
It's also possible to create your own menu and call card actions from it. Use unitRequestCardAction
event and send inside an action you want to perform.
Possible actions: Activate
, Freeze
, Unfreeze
, Report
(for report lost / stolen), Close
, Replace
, ManagePin
, ChangePin
, SetPin
, AddToWallet
Use API Docs in order to understand which actions could be applied for any particular card statuses.
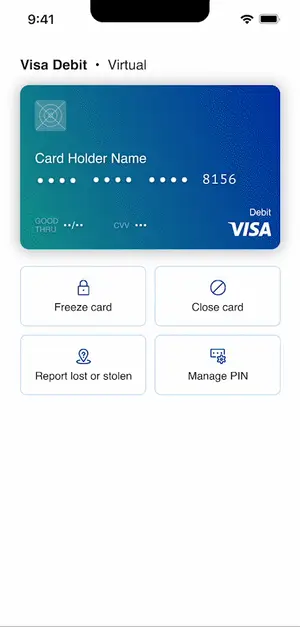
<unit-elements-card
hide-actions-menu-button="false"
hide-card-title="false"
hide-sensitive-data-button="false"
card-id="632197"
>
</unit-elements-card>
<button id="#custom-button">Freeze card</button>
<script>
document.querySelector("#custom-button").addEventListener("click", () => {
document.querySelector("unit-elements-card").dispatchEvent(
new CustomEvent("unitRequestCardAction", {
detail: { action: "Freeze" },
})
);
});
</script>
Book Payment Component
Implementation
Add the book payment element to your app where you'd like it to be presented.
<unit-elements-book-payment
account-id="1105561"
counterparty-name="Peter Parker"
counterparty-account-id="1105562"
is-same-customer="true"
theme=""
customer-token=""
initial-stage-back-button="true"
final-stage-done-button="true"
></unit-elements-book-payment>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | Yes | Unit account id. The account from which money is being sent. |
counterparty-account-id | string | No | Unit account id. The account which will receive money. |
counterparty-name | string | No | Name of the counterparty. This is the name that will be displayed in the Book Payment UI during the transfer. |
is-same-customer | boolean string ("true" / "false") | No | Stating whether both accounts belong to the same customer. Allows fetching additional information about the counterparty account. Default "false" |
is-auto-focus | boolean string ("true" / "false") | No | Auto-focus the money input field once the component is mounted. Default "false" |
customer-token | string | Yes | A Unit Customer token. Required Scopes: payments payments-write accounts |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
Events:
Event Name | Description | Detail |
---|---|---|
unitPaymentCreated | Occurs when a book payment is created | Book Payment |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |
ACH Credit Component
Implementation
Add the ACH Credit Payment Element to your app where you'd like it to be presented.
<unit-elements-ach-credit-payment
theme=""
fee="1.5"
customer-token=""
account-id="1105561"
is-auto-focus="true"
with-plaid="false"
initial-stage-back-button="true"
final-stage-done-button="true"
></unit-elements-ach-credit-payment>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts payments payments-write counterparties counterparties-write |
account-id | string | No | Unit account id. The account from which money is being sent. |
fee | string ("1.5") | No | Bill your counterparty for his activity |
with-plaid | boolean string ("true" / "false") | No | Enable Plaid integration to connect your users’ financial accounts using the Plaid API. To utilize this integration, you will need to have a Plaid account and provide your Plaid credentials through the Unit Sandbox Dashboard and Unit Production Dashboard. Default "false". |
is-auto-focus | boolean string ("true" / "false") | No | Auto-focus the 'add new recipient' button once the component is mounted. Default "false". |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
same-day | boolean string ("true" / "false") | No | Enables Same Day ACH |
plaid-account-filters | string "checking, savings" | No | Account subtypes to display in Link. If not specified, only checking subtype will be shown. |
plaid-link-customization-name | string | No | The name of the Link customization from the Plaid Dashboard to be applied to Link. If not specified, the default customization will be used. When using a Link customization, the language in the customization must match the language selected via the language parameter. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
Events:
Event Name | Description | Detail |
---|---|---|
unitPaymentCreated | Occurs when ACH payment is created | ACH payment to linked counterparty ACH payment to inline counterparty |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |
ACH Debit Component
Implementation
Add the ACH Debit Payment Element to your app where you'd like it to be presented.
The ACH Debit component is using Plaid to connect to the user's bank account.
In order to use this component, you need to have a Plaid account and provide your Plaid credentials via Unit Sandbox Dashboard and Unit Production Dashboard.
The Plaid interface can be displayed in any of Plaid's supported languages by using the language config's "local" attribute (Default: "en").
<unit-elements-ach-debit-payment
theme=""
fee="1.5"
customer-token=""
account-id="1105561"
is-auto-focus="true"
initial-stage-back-button="true"
final-stage-done-button="true"
></unit-elements-ach-debit-payment>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts payments payments-write counterparties counterparties-write |
account-id | string | No | Unit account id. The account from which money is being sent. |
fee | string ("1.5") | No | Bill your counterparty for his activity |
is-auto-focus | boolean string ("true" / "false") | No | Auto-focus the 'add new recipient' button once the component is mounted. Default "false" |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
same-day | boolean string ("true" / "false") | No | Enables Same Day ACH |
plaid-account-filters | string "checking, savings" | No | Account subtypes to display in Link. If not specified, only checking subtype will be shown. |
plaid-link-customization-name | string | No | The name of the Link customization from the Plaid Dashboard to be applied to Link. If not specified, the default customization will be used. When using a Link customization, the language in the customization must match the language selected via the language parameter. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
Events:
Event Name | Description | Detail |
---|---|---|
unitPaymentCreated | Occurs when ACH payment is created | ACH payment to inline counterparty |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |
Activity Component
Implementation
Add the activity element to your app where you'd like it to be presented.
<unit-elements-activity
account-id="1105561"
customer-token=""
theme=""
query-filter="filter[since]=2023-01-01T00:00:00.000Z&filter[until]=2023-04-26T00:00:00.000Z&sort=-createdAt"
hide-title="false"
hide-filter-button="false"
hide-back-to-top="false"
></unit-elements-activity>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | No | Unit account id. The account for which the activity will be shown. If not specified: the activity of the customer (all accounts) will be shown |
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers transactions authorizations accounts payments check-deposits |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
query-filter | string | No | Query for filtering transactions and authorizations: Transactions, Authorizations |
hide-title | string | No | Hide the component title in case value is 'true'. |
hide-filter-button | string | No | Hide filter button in case value is 'true'. |
columns | string | No | Comma separated keys to display as table headers. The default is: "summary,type,amount" |
pagination-type | string | No | Defines the method by which additional content is loaded. Possible values: "infiniteScroll" (default), "pagination" |
transactions-per-page | string | No | Number of transactions to fetch on each page or scroll to bottom. Also acts as initial number of transactions to fetch. The default is "8" for pagination and "15" for infinite scroll |
hide-back-to-top | string | No | Hide back to top button in case value is 'true'. |
Events:
Coming soon
Account Component
Implementation
Add the Account Element to your app where you'd like it to be presented.
<unit-elements-account
account-id="1105561"
customer-token=""
theme=""
hide-actions-menu-button="false"
hide-selection-menu-button="false"
menu-items="details,statements,bankVerification"
></unit-elements-account>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | No | Unit account id. The account to show, when not provided, one of the customer's accounts will be shown. |
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers statements accounts |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
hide-actions-menu-button | string | No | Hide actions menu button in case value is 'true'. |
hide-selection-menu-button | string | No | Hide selection menu button in case value is 'true'. |
show-left-to-spend | string | No | Show amount left to spend in case value is 'true', only relevant for credit accounts. |
menu-items | string | No | A list of actions, The menu dynamically adjusts based on the provided list details, statements, bankVerification . |
Events:
Event Name | Description | Detail |
---|---|---|
unitAccountChanged | Occurs when a user switches to a different account | [Promise] Account |
unitRequestLeftToSpendDetails | Occurs when a user requests left to spend details | [Promise] Account |
Incoming events:
In some cases, the default menu button won't fit into the design of an application. By using the unitRequestOpenActionsMenu
incoming event, it's possible to open the account actions bottom sheet from a custom button
<unit-elements-account
account-id="1105561"
customer-token=""
theme=""
hide-actions-menu-button="false"
hide-selection-menu-button="false"
></unit-elements-account>
<button id="#custom-button">Account Actions</button>
<script>
document.querySelector("#custom-button").addEventListener("click", () => {
document
.querySelector("unit-elements-account")
.dispatchEvent(new CustomEvent("unitRequestOpenActionsMenu"));
});
</script>
It's also possible to create your own menu and call account actions from it. Use unitRequestAccountAction
event and send inside an action you want to perform.
Possible actions: OpenAccountStatements
, OpenAccountDetails
, DownloadBankVerificationLetter
<unit-elements-account
account-id="1105561"
customer-token=""
theme=""
hide-actions-menu-button="false"
hide-selection-menu-button="false"
></unit-elements-account>
<button id="#custom-button">Download bank verification letter</button>
<script>
document.querySelector("#custom-button").addEventListener("click", () => {
document.querySelector("unit-elements-account").dispatchEvent(
new CustomEvent("unitRequestAccountAction", {
detail: { action: "DownloadBankVerificationLetter" },
})
);
});
</script>
CheckDeposit Component
Implementation
Add the check-deposit element to your app where you'd like it to be presented.
<unit-elements-check-deposit
account-id="1105561"
fee="1.5"
customer-token=""
theme=""
initial-stage-back-button="true"
final-stage-done-button="true"
></unit-elements-check-deposit>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | Yes | Unit account id. The account to deposit to. |
fee | string | No | Fee changed for making the check deposit, will be presented to the user. |
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts check-deposits |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
Events:
Event Name | Description | Detail |
---|---|---|
unitCheckDepositCreated | Occurs when a check deposit is successfully created | [Promise] CheckDeposit |
unitCheckDepositRestartRequest | Occurs when "Deposit another check" is clicked | [Promise] CheckDeposit |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |
MultipleCards Component
Implementation
Add the multiple-cards element to your app where you'd like it to be presented.
<unit-elements-multiple-cards
customer-token=""
theme=""
language=""
hide-card-actions-button="false"
disable-card-click="true"
disable-account-click="true"
columns="details,fullName,account,type,expirationDate,status"
query-filter="filter[status][0]=Stolen&filter[status][1]=Lost&filter[status][2]=ClosedByCustomer"
pagination-type="infiniteScroll"
hide-back-to-top="false"
></unit-elements-multiple-cards>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers cards cards-write |
columns | string | No | Comma separated keys to display as table headers. The default is: "details,fullName,account,type,expirationDate,status" |
pagination-type | string | No | Defines how more content is loaded. Possible values: "infiniteScroll" (default), "pagination" |
cards-per-page | string | No | Number of cards to fetch on each page or scroll to bottom. Also acts as initial number of cards to fetch. The default is "8" for pagination and "15" for infinite scroll |
hide-card-actions-button | string | No | When true, hide the card actions button that would appear on row hover. Possible values: "true" / "false" (default) |
disable-card-click | string | No | When true, will not publish a unitMultipleCardsCardClicked event when a row is clicked. Possible values: "true" / "false" (default) |
disable-account-click | string | No | When true, will not publish a unitMultipleCardsAccountClicked event when a row is clicked. Possible values: "true" / "false" (default) |
language | string | No | A URL that specifies the language configuration. |
theme | string | No | A URL that specifies the UI configuration. |
query-filter | string | No | Query for filtering cards: Cards |
hide-title | string | No | Hide the component title in case value is 'true'. |
hide-back-to-top | string | No | Hide back to top button in case value is 'true'. |
Events:
Event Name | Description | Detail |
---|---|---|
unitMultipleCardsCardClicked | Occurs when a card row is clicked | [Promise] Card |
unitMultipleCardsAccountClicked | Occurs when an account is clicked | [Promise] Account |
NextRepayment Component
Implementation
Add the next-repayment element to your app where you'd like it to be presented.
<unit-elements-next-repayment
customer-token=""
theme=""
language=""
account-id="1111111"
hide-title="false"
></unit-elements-next-repayment>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts |
account-id | string | Yes | Unit account id. The credit account for which to display next repayment. |
language | string | No | A URL that specifies the language configuration. |
theme | string | No | A URL that specifies the UI configuration. |
hide-title | string | No | Hide the component title in case value is 'true'. |
ProgramDetails Component
Implementation
Add the program-details element to your app where you'd like it to be presented.
<unit-elements-program-details
customer-token=""
theme=""
language=""
account-id="1111111"
hide-title="false"
></unit-elements-program-details>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts |
account-id | string | Yes | Unit account id. The credit account for which to display program details. |
language | string | No | A URL that specifies the language configuration. |
theme | string | No | A URL that specifies the UI configuration. |
hide-title | string | No | Hide the component title in case value is 'true'. |
Payee management Component
Implementation
Add the payee management element to your app where you'd like it to be presented.
<unit-elements-payee-management
customer-token=""
theme=""
language=""
></unit-elements-payee-management>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
customer-token | string | Yes | A Unit Customer token. Required Scopes: counterparties counterparties-write |
language | string | No | A URL that specifies the language configuration. |
theme | string | No | A URL that specifies the UI configuration. |
columns | string | No | Comma separated keys to display as table headers. The default is: "name,bank,routing,account,type". |
menu-items | string | No | A list of actions, The menu dynamically adjusts based on the provided list sendFunds, delete. |
query-filter | string | No | Query for filtering ccounterparties: Counterparties. |
pagination-type | "infiniteScroll", "pagination" | No | defines the method by which additional content is loaded. Possible values: "infiniteScroll" (default), "pagination" |
plaid-account-filters | "checking, savings" | No | Account subtypes to display in Link. If not specified, only checking subtype will be shown. |
plaid-link-customization-name | string | No | The name of the Link customization from the Plaid Dashboard to be applied to Link. If not specified, the default customization will be used. When using a Link customization, the language in the customization must match the language selected via the language parameter. |
counterparties-per-page | string | No | Number of counterparties to fetch on each page or scroll to bottom. Also acts as initial number of counterparties to fetch. The default is "8" for pagination and "15" for infinite scroll |
hide-title | "true", "false" | No | Hide the component title in case value is "true". |
hide-counterparty-actions-button | "true", "false" | No | Hide the component actions button in case value is "true". |
menu-placeholder | string | No | The ID name of the menu HTML element allows the user to render the counterparty menu in a different location. |
flow-placeholder | string | No | The ID name of the flow HTML element allows the user to render the counterpaty flow (delete, send funds) in a different location. |
payee-creation-placeholder | string | No | The ID name of the payee-creation HTML element allows the user to render the payee creation in a different location. |
micro-deposit-connection-placeholder | string | No | The ID name of the micro-deposit-connection HTML element allows the user to render the micro-deposit-connection in a different location. |
Events:
Event Name | Description | Detail |
---|---|---|
unitCounterpartyCreated | Occurs when a new counterparty created | [Promise] Counterparty |
unitCounterpartyDeleted | Occurs when selected counterparty deleted | [Promise] Counterparty |
unitConnectedAccountCreated | Occurs when a new connected account created | [Promise] ConnectedAccount |
unitMicroDepositConnectionCreated | Occurs when micro-deposit connection created | [Promise Counterparty] |
unitMicroDepositConnectionRejected | Occurs when micro-deposit connection rejected | [Promise] ConnectedAccount |
Wire Payment Component
Wire payments are not enabled by default, and are subject to a minimum payment amount that is determined by the partner bank. See docs about Wires.
Implementation
The component is performing MFA on every wire payment, the customer-token that is being used when using the component must have the upgradableScope
attribute with the wire-payments-write
scope.
An example of creating a token:
{
"data": {
"type": "customerToken",
"attributes": {
"scope": "payments accounts",
"upgradableScope": "wire-payments-write",
"verificationToken": "i8FWKLBjXEg3TdeK93G3K9PKLzhbT6CRhn/VKkTsm....",
"verificationCode": "203130"
}
}
}
Add the wire payment element to your app where you'd like it to be presented.
<unit-elements-wire-payment
account-id="1105561"
theme=""
customer-token=""
initial-stage-back-button="true"
final-stage-done-button="true"
></unit-elements-wire-payment>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | Yes | Unit account id. The account from which money is being sent. |
is-auto-focus | boolean string ("true" / "false") | No | Auto-focus the money input field once the component is mounted. Default "false" |
customer-token | string | Yes | A Unit Customer token. Required Scopes: payments accounts Required Upgradable Scopes: wire-payments-write |
fee | string | No | Fee changed for making the wire payment, will be presented to the user. |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
Events:
Event Name | Description | Detail |
---|---|---|
unitPaymentCreated | Occurs when a book payment is created | Wire Payment |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |