iOS SDK
Requirements
Name | Version |
---|---|
iOS | >= 14.0 |
Installation
Swift Package Manager
- Add the package dependency to your project: Navigate to File > Swift Packages > Add Package Dependency.
- Enter the package repository URL:
https://github.com/unit-finance/unit-ios-components-pod.git
. - Specify the version: 2.0.0
- Add the package to your target.
Cocoapods
- Set the dependency in your
Podfile
as follows:pod 'UnitComponents', '2.0.0'
- Execute
pod install
in Terminal.
Usage
Unit SDK Manager class
In order to interact with Unit's SDK, use the UnitComponentsSDK.manager
class:
let manager = UnitComponentsSDK.manager
Initialize SDK
To begin using the SDK, you must first initialize it using the initialize
function.
You may utilize the UNComponentsSDKConfigurationBuilder
. This builder allows you to set essential parameters such as the environment and webVersioningStrategy.
Usage Example:
let unitSdkManager = UnitComponentsSDK.manager
let configurationSettings = UNComponentsSDKConfigurationBuilder()
.environment(UNComponentsEnvironment.sandbox)
unitSdkManager.initialize(with: configurationSettings)
Important Note: To utilize the SDK, you must configure a customer token. It is essential to note that the customer token is not included in the initial SDK setup because it may need to be updated periodically. Please refer to the Setup SDK Parameters section for more details.
Environment
The UNComponentsEnvironment
enum should be used:
public enum UNComponentsEnvironment {
case sandbox
case production
}
Web SDK Versioning Strategy Guide
It's essential to understand that this SDK utilizes the web SDK views for certain components.
To give you optimal flexibility in managing the Web SDK versions, we've devised a strategy that allows you to either keep your SDK up-to-date or fixate on a particular version.
To set your preferred versioning approach, utilize the enum UNWebVersioningStrategy
. Below are the options you can select from:
Exact Versioning -
.exact(major: 2, minor: 4, patch: 0)
- This method allows you to lock onto a specific version that suits your needs.
Up to Next Minor -
.upToNextMinor(major: 2, minor: 4)
- This is the default and recommended approach. While it keeps your SDK updated with minor patches, a manual update is needed for minor version changes.
Up to Next Major -
.upToNextMajor(major: 2)
- With this strategy, your SDK will automatically update until there's a major version change.
Latest -
.latest
- With this strategy, your SDK will use the latest web SDK.
You can configure the web versioning strategy through the initialize
method using the UNComponentsSDKConfigurationBuilder
described above.
let configurationSettings = UNComponentsSDKConfigurationBuilder()
.environment(UNComponentsEnvironment.sandbox)
.webVersioningStrategy(UNWebVersioningStrategy.latest)
Setup SDK parameters
Customer Token
Set your customer token as follows:
manager.customerToken = "token"
Setup UI parameters
Setting Manager Properties During Initialization
It is essential to note that the manager's setters should only be invoked during the initial phase of your application. This approach aims to eliminate the need for your application to wait for resource loading, thereby enhancing operational efficiency.
Parameters that have a global impact, such as the theme JSON or fonts, should be exclusively set during the initialization of our SDK. Failure to adhere to this guideline may result in inconsistent behavior throughout the application.
In certain cases, it may be permissible to define new themes or other parameters at a component level. Such alterations can be accomplished by providing the requisite parameters as arguments when initializing the specific component.
In particular, the best practice is to define it in the AppDelegate
file, as follow:
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
let manager: UNComponentsManagerProtocol = UnitComponentsSDK.manager
manager.environment = .sandbox
manager.customerToken = customerToken
return true
}
Theme
The theme is a URL that specifies the UI configuration. Set the theme as follows:
manager.ui.setTheme("https://url.com")
Language
The language is a URL that specifies the language configuration. Set the language as follows:
manager.ui.setLanguage("https://url.com")
For the latest SDK, the latest webVersioningStrategy is upToNextMinor(major: 1, minor: 6)
.
Fonts
Add the Font File to Your Project
- Locate the
.ttf
or.otf
font file you wish to add. - Drag and drop the font file into your Xcode project. Make sure to check the "Copy items if needed" option and add it to your desired target.
Edit Info.plist
You need to register the custom font by adding the "Fonts provided by application" key in the Info.plist
file.
Now, You can configure custom fonts in your application by utilizing the typealias UNComponentsFonts
as shown below:
public typealias UNComponentsFonts = [UNComponentsFontFamilyName: [UNComponentsFontData]]
public typealias UNComponentsFontFamilyName = String
In this dictionary, the keys typically represent the font family names. Corresponding to each font family name, there is an array containing various fonts from that particular family.
Note: Our current implementation supports font names formatted as <font-family>-<font-weight>
.
UNComponentsFontData
Properties
Name | Type | Description |
---|---|---|
fontWeight | FontWeight | Enum that defines the weight of the font. |
sources | [UNComponentsFontSource] | An array of UNComponentsFontSource objects. An array is used to provide fallback options. |
UNComponentsFontSource
Properties
Name | Type | Description |
---|---|---|
fileName | String | The custom font file name, as specified in the info.plist of your target |
format | String? | (Optional) Font file format. Useful for specifying fallback behavior. |
Swift Code Example
manager.ui.setFonts([
"Poppins": [
UNComponentsFontData(fontWeight: FontWeight.regular, sources: [UNComponentsFontSource(fileName: "Poppins-Regular.ttf")]),
UNComponentsFontData(fontWeight: FontWeight.bold, sources: [UNComponentsFontSource(fileName: "Poppins-Bold.ttf")])
],
"Arial": [
UNComponentsFontData(fontWeight: FontWeight.regular, sources: [UNComponentsFontSource(fileName: "Arial-Regular.ttf")]),
]
])
Authentication
To ensure the security and privacy of user data when using our SDK, it is crucial to clean user data each time you logout (or switch between customers). This helps to prevent unauthorized access and data leakage.
Clean User Data
Whenever you need to switch between customers or perform a logout operation, make sure to invoke the cleanUserData
method. This method will clear all the stored user data from the session, ensuring a fresh and secure state for the new customer session.
manager.authentication.cleanUserData()
Components
All the components extend from UIView
.
Each component receives the callbacks
as an argument, the callbacks
is a typealias
of a method that receives a callback
enum and returns nothing.
The callback
enum has all of the possible callbacks that you can receive from this component.
UNComponents SDK includes the following UI components:
Component | Protocol | Callbacks Type |
---|---|---|
Card | UNCardView | UNCardComponentCallbacks |
ACH Credit | UNACHCreditView | UNACHCreditComponentCallbacks |
ACH Debit | UNACHDebitView | UNACHDebitComponentCallbacks |
Account | UNAccountView | UNAccountComponentCallbacks |
Activity | UNActivityView | UNActivityComponentCallbacks |
BookPayment | UNBookPaymentView | UNBookPaymentComponentCallbacks |
Check Deposit | UNCheckDepositView | UNCheckDepositComponentCallbacks |
Multiple Cards | UNMultipleCardsView | UNMultipleCardsComponentCallbacks |
Program Details | UNProgramDetailsView | UNProgramDetailsComponentCallback |
Wire Payment | UNWirePaymentView | UNWirePaymentComponentCallback |
In order to access the UI components, use manager.ui.views
:
let manager = UnitComponentsSDK.manager
let unViews = manager.ui.views
Security
UNComponentsSecuritySettingsBuilder
is designed to help you configure your security settings.
Snapshot Protection
UNSnapshotProtectionStrategy
defines how to protect your app's content from being captured in snapshots. The default strategy is fullProtection(style: .light)
.
The enum offers three strategies:
- Full Protection - applies protection to all screens within the application.
- Views - applies protection only to Unit views.
- None - no protection applied (likely used if you implement your own protection).
Example:
let snapshotStrategy = UNSnapshotProtectionStrategy.fullProtection(style: .light)
unitSdkManager.securitySettings = UNComponentsSecuritySettingsBuilder()
.snapshotProtectionStrategy(snapshotStrategy)
Set redirect-uri for Plaid
In order to use Plaid, you must set redirectUri (so we can create token correctly as explained in Plaid documentation).
The redirect uri must match the one set in Plaid dashboard with /plaid
suffix. For example, if the redirect-uri set on Plaid dashboard is https://your.example.domain/plaid
, then the redirect-uri set to UnitManager must be https://your.example.domain
.
Example:
import UIKit
import UNComponents
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
...
let manager: UNComponentsManagerProtocol = UnitComponentsSDK.manager
manager.helpers.setRedirectUri("https://your.example.domain")
return true
}
...
}
Error Handling
By using unitOnLoad callback you can get a Swift. Result about the requested component. OnError - you will get an enum UNComponentsError that consists of several cases.
UNComponentsError:
Case | fields | Description |
---|---|---|
onLoad | errors: [UNComponentsErrorResponse] | Report UNComponentsErrorResponse on errors that happen during loading time |
UNComponentsErrorResponse:
Name | Type | Description |
---|---|---|
status | String | A Unit-specific code, uniquely identifying the error type |
title | String? | The title of the error type |
detail | String? | Additional information about the error. |
details | String? | Additional information about the error. |
meta | Meta?: { supportId: String } | meta.supportId unique error identifier to be used for further investigation |
Note: An "error object" MUST contain a title and the HTTP status code members.
Example:
fileprivate lazy var accountComponent: UNAccountView = {
let unViews = UnitComponentsSDK.manager.ui.views
let accountComponent = unViews.getAccountComponent(accountId: "424242"){ callback in
switch callback {
case .unitOnLoad(let result):
switch result {
case .success(let accounts):
print("Success. Accounts Data: \(accounts)")
case .failure(let error):
switch error {
case .onLoad(let onLoadErrors):
print("Fail to load Account component. Errors:")
for loadError in onLoadErrors {
print("Status: \(loadError.status); Title:\(loadError.title)")
}
}
}
}
return accountComponent
}()
Components working together
Unit Components can effectively communicate with each other. They can automatically exchange messages and trigger events to update relevant data in other components.
For instance, the Payment components and Activity components can work together such that, after a payment has been processed, the new transactions are automatically displayed on the Activity component.
Similarly, the Payment components and Account components can communicate such that, after a payment has been made, the account balance is updated on the Account component.
In some cases, a component may need to be updated in response to an event that is triggered by another component.
For example, if a customer has multiple accounts, the app may have an Account component and an Activity component that displays the activity of a selected account.
let unViews = UnitComponentsSDK.manager.ui.views
fileprivate lazy var activityComponent: UNActivityView = {
let activityComponent = unViews.getActivityComponent()
return activityComponent
}()
fileprivate lazy var accountComponent: UNAccountView = {
let accountComponent = unViews.getAccountComponent() { callback in
switch callback {
case .onAccountChange(let account):
// Update the account for the activity component
self.activityComponent.accountId = account.id
default:
break
}
}
return accountComponent
}()
The Account component has an account switcher that can change the current selected account.
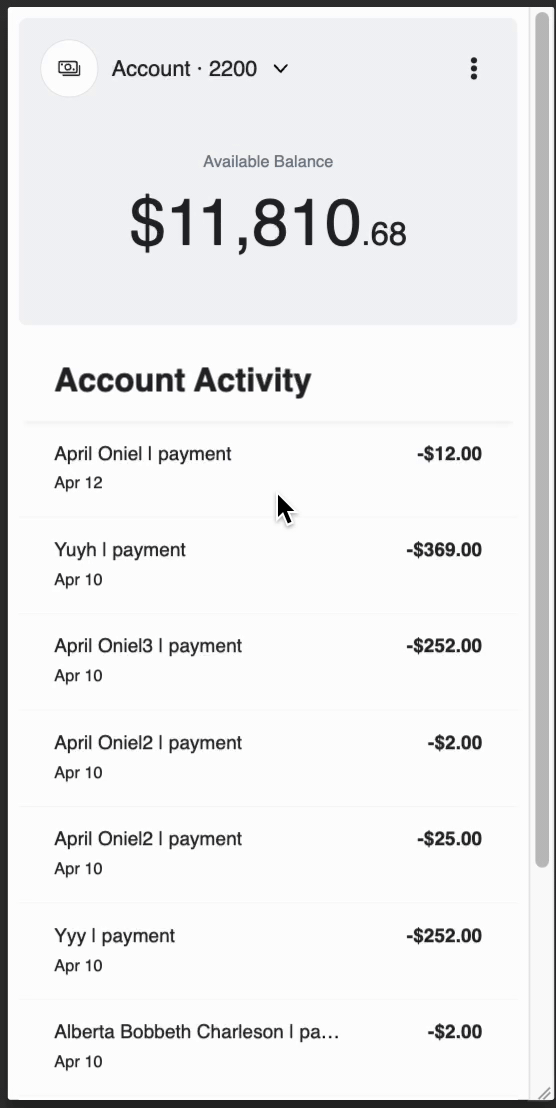