Check Deposits
The check deposit component allows you to let your customers deposit a check into a specific deposit account. The component takes the user through all the required stages:
- Set amount
- Take photos of the front and back of the check
- Review and submit the check deposit
Implementation
Web Components
Add the check-deposit element to your app where you'd like it to be presented.
<unit-elements-check-deposit
account-id="1105561"
fee="1.5"
customer-token=""
theme=""
initial-stage-back-button="true"
final-stage-done-button="true"
enable-device-handoff="true"
></unit-elements-check-deposit>
Inputs:
Name | Type | Required | Description |
---|---|---|---|
account-id | string | Yes | Unit account id. The account to deposit to. |
fee | string | No | Fee charged for making the check deposit, will be presented to the user. |
customer-token | string | Yes | A Unit Customer token. Required Scopes: customers accounts check-deposits check-deposits-write |
theme | string | No | A URL that specifies the UI configuration. |
language | string | No | A URL that specifies the language configuration. |
initial-stage-back-button | boolean string ("true" / "false") | No | An action button at the first stage of the payment flow. Default "false" |
final-stage-done-button | boolean string ("true" / "false") | No | An action button at the final stage of the payment flow. Default "false" |
enable-device-handoff | boolean string ("true" / "false") | No | If set to true, will allow handoff to mobile device from Desktop. |
Events:
Event Name | Description | Detail |
---|---|---|
unitCheckDepositCreated | Occurs when a check deposit is successfully created | [Promise] CheckDeposit |
unitCheckDepositRestartRequest | Occurs when "Deposit another check" is clicked | [Promise] CheckDeposit |
unitPaymentInitialStageBackButtonClicked | Occurs when the initial stage back button is clicked | |
unitPaymentFinalStageDoneButtonClicked | Occurs when the final stage done button is clicked |
Device handoff:
Check deposit component supports device handoff feature, which allows users to start the check deposit process on a desktop device and continue on a mobile device. By scanning a QR code, user will be redirected to the mobile device with the check deposit component.
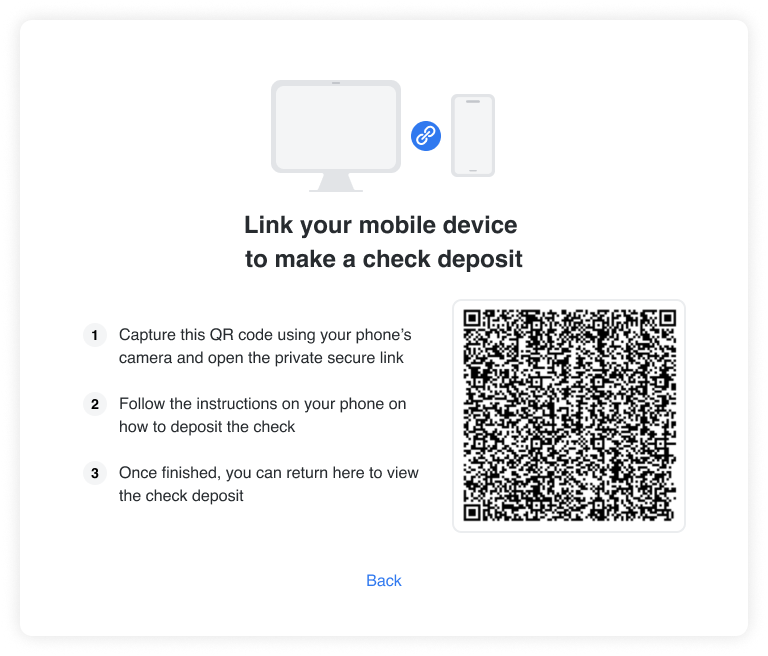
React Native SDK
Prerequirements:
To enable check scanning with our SDK, please request the camera permissions in your application.
Android
: navigate to the AndroidManifest.xml
file and insert the following:
<uses-permission android:name="android.permission.CAMERA" />
iOS
: navigate to the Info.plist
file and insert the camera permission key:
<key>NSCameraUsageDescription</key>
<string>Your custom message requesting permission from the user</string>
UNCheckDeposit props:
Name | Type | Required | Description |
---|---|---|---|
accountId | string | YES | Unit account id. The account to deposit to. |
fee | number | NO | Fee changed for making the check deposit, will be presented to the user. |
customerToken | string | YES | A Unit Customer token. |
theme | string | NO | A URL that specifies the UI configuration. |
language | string | NO | A URL that specifies the language configuration. |
initialStageBackButton | boolean | NO | An action button at the first stage of the payment flow. Default false |
finalStageDoneButton | boolean | NO | An action button at the final stage of the payment flow. Default false |
onInitialStageBackButtonClicked | () => Void | NO | Occurs when the initial stage back button is clicked. |
onFinalStageDoneButtonClicked | () => Void | NO | Occurs when the final stage done button is clicked. |
onDepositCreated | (depositCheckData: UNCheckDeposit) => Void | NO | Occurs when a check deposit is successfully created |
onRestartRequest | (depositCheckData: UNCheckDeposit) => Void | NO | Occurs when "Deposit another check" is clicked |
onLoad | (response: UNOnLoadResponse<UNAccountData>) => void | NO | Callback for a loaded component |
Example:
import React from 'react';
import {
UNCheckDepositComponent,
UNCheckDepositData,
UNAccountData,
UNOnLoadResponse,
} from 'react-native-unit-components';
export default function YourComponent() {
return (
<UNCheckDepositComponent
customerToken={'<Your customer token>'}
accountId={'424242'}
fee={1.5}
onDepositCreated={(checkData: UNCheckDepositData) =>
console.log('Check deposit created:', checkData)
}
onRestartRequest={(checkData: UNCheckDepositData) =>
console.log('Restart Request. Check data: ', checkData)
}
onLoad={(response: UNOnLoadResponse<UNAccountData>) => {
console.log(response);
}}
/>
);
}
Android SDK
Component name: UNCheckDepositComponent
Prerequirements:
To enable check scanning with our SDK, please request the camera permissions in your application manifest.
<uses-permission android:name="android.permission.CAMERA" />
getCheckDepositComponent parameters:
Name | Type | Required | Description |
---|---|---|---|
accountId | String | YES | Unit account id. |
additionalSettings | UNCheckDepositViewSettingsInterface | NO | Additional settings unique for this component |
theme | String | NO | A URL that specifies the UI configuration. |
language | String | NO | A URL that specifies the language configuration. |
callbacks | UNCheckDepositComponentCallbacks | NO | Component's Callbacks sealed class |
UNCheckDepositComponentCallbacks
The callbacks parameter for UNAccountComponent is of the following type:
typealias UNCheckDepositComponentCallbacks = (callback: UNCheckDepositComponentCallback) -> Unit
The UNCheckDepositComponentCallback is sealed class that has the following callbacks that you can receive from a CheckDeposit component:
sealed class UNCheckDepositComponentCallback {
data object OnInitialStageBackButtonClicked: UNCheckDepositComponentCallback()
data object OnFinalStageDoneButtonClicked: UNCheckDepositComponentCallback()
data class OnDepositCreated(val checkDepositData: UNCheckDepositData): UNCheckDepositComponentCallback()
data class OnRestartRequest(val checkDepositData: UNCheckDepositData): UNCheckDepositComponentCallback()
data class OnLoad(val onLoadResponse: Result<UNAccountData>): UNCheckDepositComponentCallback()
}
To get the CheckDeposit Component fragment, call the getCheckDepositComponent
method of UnitComponentsSdk.manager.ui.views
.
Example:
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.LinearLayout
import androidx.fragment.app.Fragment
import co.unit.un_components.api.UnitComponentsSdk
import co.unit.un_components.common.builders.UNCheckDepositViewSettingsBuilder
import co.unit.un_components.common.enums.UNCheckDepositComponentCallback
import co.unit.un_components.components.UNCheckDepositView
class CheckDepositFragment : Fragment(){
override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
private var unCheckDepositComponent: UNCheckDepositComponent? = null
unCheckDepositComponent = UnitComponentsSdk.manager.ui.views.getCheckDepositComponent(
accountId = YOUR_ACCOUNT_ID,
theme = YOUR_THEME_URL,
language = YOUR_LANGUAGE_URL
) { callback ->
when(callback) {
is UNCheckDepositComponentCallback.OnInitialStageBackButtonClicked -> handleInitialStageBackButtonClicked()
is UNCheckDepositComponentCallback.OnFinalStageDoneButtonClicked -> handleFinalStageDoneButtonClicked()
is UNCheckDepositComponentCallback.OnDepositCreated -> handleDepositCreated(callback.checkDepositData)
is UNCheckDepositComponentCallback.OnRestartRequest -> handleRestartRequest(callback.checkDepositData)
is UNCheckDepositComponentCallback.OnLoad -> handleOnLoad(callback.onLoadResponse)
}
}
val fragmentLayout = inflater.inflate(R.layout.FILE_NAME, container, false)
childFragmentManager.beginTransaction()
.replace(R.id.CONTAINER_NAME, unCheckDepositComponent as Fragment)
.commitNow()
return fragmentLayout
}
// ...
// Event handlers
// ...
}
The additionalSettings parameter for UNCheckDepositComponent has this attribute:
UNCheckDepositViewSettingsInterface
Name | Type | Default Value | Description |
---|---|---|---|
fee | Double | null | Fee charged for making the check deposit, will be presented to the user. |
initialStageBackButton | Boolean | false | An action button at the first stage of the payment flow. |
finalStageDoneButton | Boolean | false | An action button at the final stage of the payment flow. |
You can use UNCheckDepositViewSettingsBuilder()
to create an instance of the interface and define your additional settings.
val additionalSettings = UNCheckDepositViewSettingsBuilder()
.fee(...)
.initialStageBackButton(...)
.finalStageDoneButton(...)
unCheckDepositComponent = UnitComponentsSdk.manager.ui.views.getCheckDepositComponent(
accountId = YOUR_ACCOUNT_ID,
additionalSettings = additionalSettings,
theme = YOUR_THEME_URL,
language = YOUR_LANGUAGE_URL
)
IOS SDK
Component name: UNCheckDepositComponent
Prerequirements:
Check Deposit Component requires camera access. In order to enable this functionality, you need to include the Privacy - Camera Usage Description
key in your info.plist
file.
The value should be the text that will be displayed when the app prompts the user for camera access.
Configuration parameters:
Name | Type | Required | Description |
---|---|---|---|
accountId | String | YES | Unit account id. The account to deposit to. |
fee | Double | NO | Fee charged for making the check deposit, will be presented to the user. |
additionalSettings | UNCheckDepositViewSettingsProtocol | NO | Advanced optional settings. |
callbacks | UNCheckDepositComponentCallbacks | NO | Callbacks to interact with the Activity component. |
theme | String | NO | A URL that specifies the UI configuration. |
language | String | NO | A URL that specifies the language configuration. |
The callbacks
parameter for UNCheckDepositComponent
is of the following type:
public typealias UNCheckDepositComponentCallbacks = (_ callback: UNCheckDepositComponentCallback) -> Void
The UNCheckDepositComponentCallback
is an enum
that has the following callbacks that you can receive from a Book Payment component:
Key | Type | Description |
---|---|---|
unitCheckDepositCreated | (depositCheckData: UNCheckDeposit) => Void | Occurs when a check deposit is successfully created |
unitCheckDepositRestartRequest | (depositCheckData: UNCheckDeposit) => Void | Occurs when "Deposit another check" is clicked |
unitOnLoad | (result: Result<UNAccountData, UNError>) => Void | Callback for a loaded component |
onInitialStageBackButtonClicked | () => Void | Occurs when the back button is clicked on the initial stage |
onFinalStageDoneButtonClicked | () => Void | Occurs when the done button is clicked on the final stage |
To get the Check Deposit Component view call the getCheckDepositComponent
method of UnitSDK.manager.ui.views
.
Example:
import UIKit
import UNComponents
import SnapKit
class CheckDepositScreen: UIViewController {
fileprivate lazy var checkDepositComponent: UNCheckDepositView = {
let unViews = UnitSDK.manager.ui.views
let checkDepositComponent = unViews.getCheckDepositComponent(accountId: "424242", fee: 1.5) { callback in
switch callback {
case .onInitialStageBackButtonClicked
print("Back button clicked on the initial stage")
case .onFinalStageDoneButtonClicked
print("Done button clicked on the final stage")
case .unitCheckDepositCreated(let checkData):
print("Check deposit created, data: \(checkData)")
case .unitCheckDepositRestartRequest(let checkData):
print("Check deposit restart request \(checkData)")
case .unitOnLoad(let accountData):
print("CheckDeposit Component is loaded. Account: \(accountData)")
}
}
return checkDepositComponent
}()
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
view.addSubview(checkDepositComponent)
checkDepositComponent.snp.makeConstraints { make in
make.top.equalTo(view.safeAreaLayoutGuide.snp.top)
make.bottom.equalTo(view.safeAreaLayoutGuide.snp.bottom)
make.leading.equalTo(view.safeAreaLayoutGuide.snp.leading)
make.trailing.equalTo(view.safeAreaLayoutGuide.snp.trailing)
}
}
}
The additionalSettings
parameter for UNCheckDepositComponent
is of the following type:
UNCheckDepositViewSettingsProtocol:
Name | Type | Default Value | Description |
---|---|---|---|
initialStageBackButton | Bool | false | An action button at the first stage of the payment flow. |
finalStageDoneButton | Bool | false | An action button at the final stage of the payment flow. |
You can use UNCheckDepositViewSettingsBuilder
to create an instance of the protocol and define your additional settings.
Example:
let additionalSettings = UNCheckDepositViewSettingsBuilder()
.initialStageBackButton(true)
.finalStageDoneButton(true)
let unViews = UnitSDK.manager.ui.views
let checkDepositComponent = unViews.unViews.getCheckDepositComponent(accountId: "424242", fee: 1.5, additionalSettings: additionalSettings)