Android SDK
Installation
Add the following repository to the project settings.gradle
file inside dependencyResolutionManagement
repositories:
maven {
url "https://nexus.i.unit.co/repository/maven/"
}
Add the un-components dependency to your project:
dependencies {
implementation 'co.unit:un-components:1.0.0'
}
Usage
Unit SDK Manager class
In order to interact with Unit's SDK, use the UnitComponentsSdk.manager
class:
val manager = UnitComponentsSdk.manager
Initialize SDK
To begin using the SDK, you must first initialize it using the initialize
function.
You may utilize the UNComponentsInitializationSettingsBuilder
. This builder allows you to set essential parameters such as the environment and webVersioningStrategy.
val unitSdkManger = UnitComponentsSdk.manager
val initializationSettings = UNComponentsInitializationSettingsBuilder()
.environment(UNComponentsEnvironment.Sandbox)
.webVersioningStrategy(UNWebVersioningStrategy.Latest)
unitSdkManger.initialize(initializationSettings)
It is recommended to call it in onCreate
method of your main activity.
Important Note: To utilize the SDK, you must configure a customer token. It is essential to note that the customer token is not included in the initial SDK setup because it may need to be updated periodically. Please refer to the Setup SDK Parameters section for more details.
Environment
The UNComponentsEnvironment
enum class should be used:
enum class UNComponentsEnvironment {
Sandbox,
Production,
Mock
}
Web SDK Versioning Strategy Guide
It's essential to understand that this SDK utilizes the web SDK views for certain components.
To give you optimal flexibility in managing the Web SDK versions, we've devised a strategy that allows you to either keep your SDK up-to-date or fixate on a particular version.
To set your preferred versioning approach, utilize the sealed class UNWebVersioningStrategy
. Below are the options you can select from:
Exact Versioning -
data class Exact(val major: Int, val minor: Int, val patch: Int)
- This method allows you to lock onto a specific version that suits your needs.
Up to Next Minor -
data class UpToNextMinor(val major: Int, val minor: Int)
- This is the default and recommended approach. While it keeps your SDK updated with minor patches, a manual update is needed for minor version changes.
Up to Next Major -
data class UpToNextMajor(val major: Int)
- With this strategy, your SDK will automatically update until there's a major version change.
Latest -
object Latest
- This strategy will always keep your SDK updated with the latest version.
For a comprehensive understanding, refer to our Web SDK - Versioning Guide.
You can configure the web versioning strategy through the initialize
method using the UNComponentsInitializationSettingsBuilder
described above.
val initializationSettings = UNComponentsInitializationSettingsBuilder()
.environment(UNComponentsEnvironment.Sandbox)
.webVersioningStrategy(UNWebVersioningStrategy.Latest)
Setup SDK parameters
Customer Token
Set your customer token as follows:
unitSdkManger.customerToken = "token"
Setup UI parameters
Setting Manager Properties During Initialization
It is essential to note that the manager's setters should only be invoked during the initial phase of your application. This approach aims to eliminate the need for your application to wait for resource loading, thereby enhancing operational efficiency.
Parameters that have a global impact, such as the theme JSON or fonts, should be exclusively set during the initialization of our SDK. Failure to adhere to this guideline may result in inconsistent behavior throughout the application.
In certain cases, it may be permissible to define new themes or other parameters at a component level. Such alterations can be accomplished by providing the requisite parameters as arguments when initializing the specific component.
In particular, the best practice is to define it in the MainActivity
file, as follow:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val manager = UnitComponentsSdk.manager
// ...
// After calling the initialize
manager.initializationSettings?.environment = UNComponentsEnvironment.Sandbox
manager.customerToken = customerToken
// ...
}
// ...
}
Theme
The theme is a URL that specifies the UI configuration. Set the theme as follows:
UnitComponentsSdk.manager.ui.theme = "https://url.com"
Language
The language is a URL that specifies the language configuration. Set the language as follows:
UnitComponentsSdk.manager.ui.language = "https://url.com"
Fonts
Add the Font File to Your Project
- Locate the
.ttf
or.otf
font file you wish to add. - Add them to your
res/font
folder in your Android Studio project. Create the font folder if it doesn't exist. In order to create the assets resource folder: right click on 'res' -> new -> folder -> Assets Folder - Add them to your
assets/fonts
folder.
Note: Android supports only alphanumeric characters and underscores in file names. Therefore, your custom font file name should adhere to this convention and may look like arial_regular.ttf
.
Now, You can configure custom fonts in your application by utilizing the typealias UNFonts
as shown below:
typealias UNFonts = Map<UNFontFamilyName, Array<UNFontData>>
typealias UNFontFamilyName = String
In this map, the keys typically represent the font family names. Corresponding to each font family name, there is an array containing various fonts from that particular family.
UNFontData
Properties
Name | Type | Description |
---|---|---|
fontResId | Int | The resource id of the font |
fontWeight | FontWeight | Enum that defines the weight of the font. |
sources | [UNFontSource] | An array of UNFontSource objects. An array is used to provide fallback options. |
Note:
It is imperative to recognize that the fontResId
should be designated as the primary font to be utilized by our SDK. The array of font sources primarily serves to offer fallback options in scenarios involving WebView usage.
UNFontSource
Properties
Name | Type | Description |
---|---|---|
fileRelativePath | String | The custom font file relative path from the project assets directory. |
format | String? | (Optional) Font file format. Useful for specifying fallback behavior. |
Kotlin Code Example
private val fonts: UNFonts = mapOf(
"Lugrasimo" to arrayOf(
UNFontData(
fontResId = R.font.lugrasimo_regular,
sources = listOf(UNFontSource(fileRelativePath = "fonts/lugrasimo/lugrasimo_regular.ttf")),
fontWeight = FontWeight.Regular,
),
UNFontData(
fontResId = R.font.lugrasimo_bold,
sources = listOf(UNFontSource(fileRelativePath = "fonts/lugrasimo/lugrasimo_bold.ttf")),
fontWeight = FontWeight.Bold,
),
),
"Arial" to arrayOf(
UNFontData(
fontResId = R.font.arial_regular,
sources = listOf(UNFontSource(fileRelativePath = "fonts/arial/arial_regular.ttf")),
fontWeight = FontWeight.Regular,
)
)
)
UnitComponentsSdk.manager.ui.fonts = fonts
Authentication
To ensure the security and privacy of user data when using our SDK, it is crucial to clean user data each time you logout (or switch between customers). This helps to prevent unauthorized access and data leakage.
Clean User Data
Whenever you need to switch between customers or perform a logout operation, make sure to invoke the cleanUserData
method. This method will clear all the stored user data from the session, ensuring a fresh and secure state for the new customer session.
UnitComponentsSdk.manager.authentication.cleanUserData()
Components
All the components extend from Fragments
.
Each component receives the callbacks
as an argument, the callbacks
is a typealias
of a method that receives a callback
sealed class and returns nothing.
The callback
sealed class has all of the possible callbacks that you can receive from this component.
UnitComponents SDK includes the following UI components:
Component | Interface | Callbacks Type |
---|---|---|
Card | UNCardComponent | UNCardComponentCallbacks |
ACH Credit | UNACHCreditComponent | UNACHCreditComponentCallbacks |
ACH Debit | UNACHDebitComponent | UNACHDebitComponentCallbacks |
Account | UNAccountComponent | UNAccountComponentCallbacks |
Activity | UNActivityComponent | UNActivityComponentCallbacks |
BookPayment | UNBookPaymentComponent | UNBookPaymentComponentCallbacks |
Check Deposit | UNCheckDepositComponent | UNCheckDepositComponentCallbacks |
Multiple Cards | UNMultipleCardsComponent | UNMultipleCardsComponentCallbacks |
Program Details | UNProgramDetailsComponent | UNProgramDetailsComponentCallback |
Wire Payment | UNWirePaymentComponent | UNWirePaymentComponentCallback |
In order to access the UI components, use manager.ui.views
:
val manager = UnitComponentsSdk.manager
val unViews = manager.ui.views
Flows
Add card to wallet flow
Start by following the Add card to wallet instructions to use this flow.
After that, you can add a card to Google Wallet by calling the Unit SDK's startPushProvisioningFlow(context: Context, cardId: String)
method.
Example:
UnitComponentsSdk.manager.ui.flows.startPushProvisioning(context, YOUR_CARD_ID)
Security
UNComponentsSecuritySettingsBuilder
is designed to help you configure your security settings.
Snapshot Protection
UNSnapshotProtectionStrategy
defines how to protect your app's content from being captured in snapshots. The default strategy is FullProtection
.
The enum offers two strategies:
- Full Protection - Protects the entire activity's views from being captured in snapshots.
- None - No protection applied (likely used if you implement your own protection).
Example:
val snapshotStrategy = UNSnapshotProtectionStrategy.FullProtection
UnitComponentsSdk.manager.securitySettings = UNComponentsSecuritySettingsBuilder().snapshotProtectionStrategy(snapshotStrategy)
Error Handling
By using unitOnLoad callback you can get a result of type Kotlin.Result for the requested component. On error - you will get a sealed class UNComponentsError that consist of several cases.
UNComponentsError:
Case | fields | Description |
---|---|---|
OnLoad | errors: ArrayList<UNComponentsErrorResponseObject> | Report UNComponentsErrorResponse on errors that happen during loading time |
UNComponentsErrorResponseObject:
Name | Type | Description |
---|---|---|
status | String | A Unit-specific code, uniquely identifying the error type. |
title | String | The title of the error type. |
detail | String? | Additional information about the error. |
details | String? | Additional information about the error. |
meta | Any? | Identifier to be used for further investigation. |
Note: An UNComponentsErrorResponseObject
MUST contain a title and the HTTP status code members.
Components working together
Unit Components can effectively communicate with each other. They can automatically exchange messages and trigger events to update relevant data in other components.
For instance, the Payment components and Activity components can work together such that, after a payment has been processed, the new transactions are automatically displayed on the Activity component.
Similarly, the Payment components and Account components can communicate such that, after a payment has been made, the account balance is updated on the Account component.
In some cases, a component may need to be updated in response to an event that is triggered by another component.
For example, if a customer has multiple accounts, the app may have an Account component and an Activity component that displays the activity of a selected account.
private val views = UnitComponentsSdk.manager.ui.views
val unActivityView = views.getActivityComponent(ACCOUNT_ID)
val unAccountView = views.getAccountComponent(ACCOUNT_ID) { callback ->
when (callback) {
is UNAccountComponentCallback.AccountChanged -> {
// Update the account for the activity component
unActivityView.accountId = callback.account.id
}
}
}
The Account component has an account switcher that can change the current selected account.
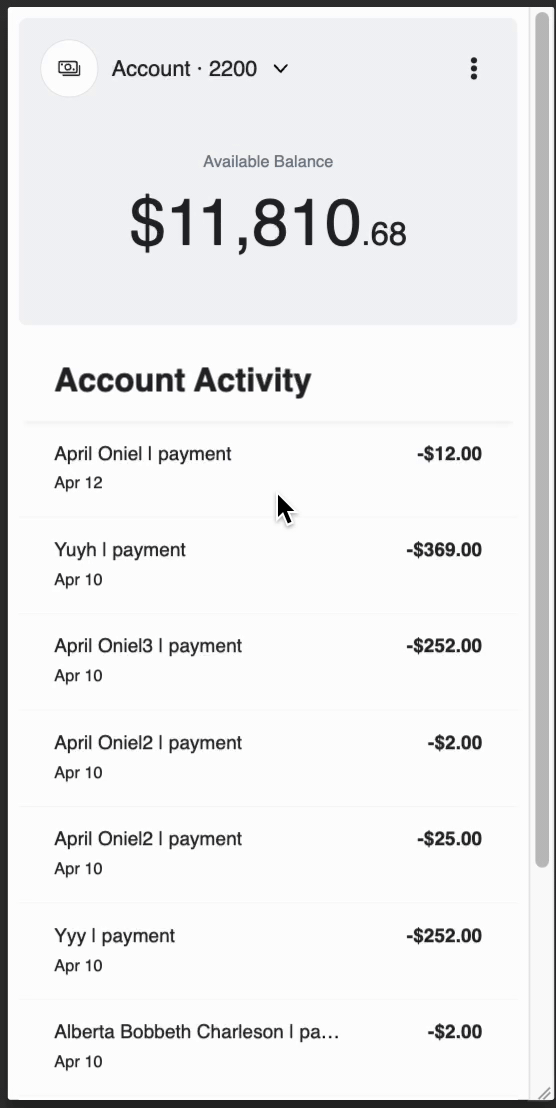